题目描述
给定一个二叉树 root
,返回其最大深度。
二叉树的 最大深度 是指从根节点到最远叶子节点的最长路径上的节点数。
示例 1:
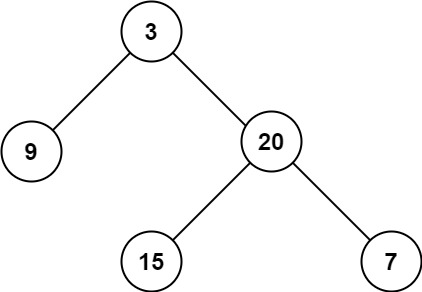
1 2
| 输入:root = [3,9,20,null,null,15,7] 输出:3
|
示例 2:
1 2
| 输入:root = [1,null,2] 输出:2
|
提示:
- 树中节点的数量在
[0, 104]
区间内。
-100 <= Node.val <= 100
题解
深度优先遍历
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23
|
class Solution { public int maxDepth(TreeNode root) { if (root == null) { return 0; } return Integer.max(maxDepth(root.left), maxDepth(root.right)) + 1; } }
|
提交:

广度优先遍历
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38
|
class Solution { public int maxDepth(TreeNode root) { Queue<TreeNode> queue = new LinkedList<>(); if (root != null) { queue.add(root); } int ans = 0; while (!queue.isEmpty()) { int size = queue.size(); ans++; for (int i = 0; i < size; i++) { TreeNode poll = queue.poll(); if (poll.left != null) { queue.add(poll.left); } if (poll.right != null) { queue.add(poll.right); } } } return ans; } }
|
提交:
